How to generate qr codes with PHP
QR codes are two-dimensional barcodes that can encode a variety of information, including text, URLs, phone numbers, and email addresses. They are becoming increasingly popular in a variety of applications, such as marketing, product packaging, and event registration.
Generating QR codes using PHP is a relatively simple process. There are a number of PHP libraries available that can be used to generate QR codes, such as Endroid QR Code and PHP QR Code.
In this article, we will learn how to generate QR codes using PHP using the Endroid QR Code library. We will also learn how to customize the appearance of the QR codes, such as size, color, and error correction level.
If your looking to simply generate a qr code and use as you will, feel free to use our Free QR code Generator.
Installing Endroid QR Code
Endroid QR Code library can be found on GitHub
To install the library, run the following command:
composer require endroid/qr-code;
Using Endroid QR Code to create a QR Code
Once the library is installed, go ahead and include it into your php file
use Endroid\QrCode\Color\Color;
use Endroid\QrCode\Encoding\Encoding;
use Endroid\QrCode\ErrorCorrectionLevel\ErrorCorrectionLevelLow;
use Endroid\QrCode\QrCode;
use Endroid\QrCode\Label\Label;
use Endroid\QrCode\Logo\Logo;
use Endroid\QrCode\RoundBlockSizeMode\RoundBlockSizeModeMargin;
use Endroid\QrCode\Writer\PngWriter;
use Endroid\QrCode\Writer\ValidationException;
The QR code I want to generate is going to be in PNG format.
Now lets take a look at the various type of QR Codes we can generate.
Generate Plain Text or URL QR Code
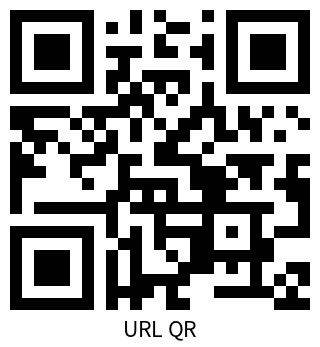
$url = 'https://codecr8.com';
$writer = new PngWriter();
// Create QR code
$qrCode = QrCode::create($url)
->setEncoding(new Encoding('UTF-8'))
->setErrorCorrectionLevel(new ErrorCorrectionLevelLow())
->setSize(300)
->setMargin(10)
->setRoundBlockSizeMode(new RoundBlockSizeModeMargin())
->setForegroundColor(new Color(0, 0, 0))
->setBackgroundColor(new Color(255, 255, 255));
$label = Label::create('Scan Me')
->setTextColor(new Color(0, 0, 0));
$logo = null;
$result = $writer->write($qrCode, $logo, $label);
$dataUri = $result->getDataUri();
The above code will generate the QR Code with a message on the bottom saying Scan Me and the response will be the base64 data. If you want to save the file locally you can add the saveToFile call.
$result->saveToFile(__DIR__.'/qrcode.png');
Generate QR Code to Send Emails
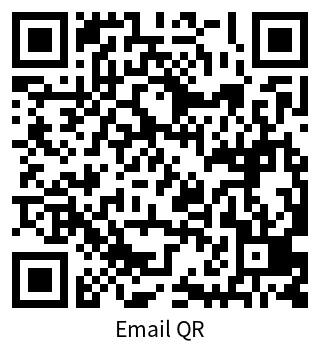
QR codes can also be used to open up the email app and have some information pre-filled for easier way of sending emails. Let’s write the code for generating a QR code that will send a email.
$qr_string = 'mailto:[email protected]?subject=QR code to Email'&body=Pre Filled Message body goes here';
// Create QR code
$qrCode = QrCode::create($qr_string)
->setEncoding(new Encoding('UTF-8'))
->setErrorCorrectionLevel(new ErrorCorrectionLevelLow())
->setSize(300)
->setMargin(10)
->setRoundBlockSizeMode(new RoundBlockSizeModeMargin())
->setForegroundColor(new Color(0, 0, 0))
->setBackgroundColor(new Color(255, 255, 255));
$label = Label::create('Email Me')
->setTextColor(new Color(0, 0, 0));
$logo = null;
$result = $writer->write($qrCode, $logo, $label);
$dataUri = $result->getDataUri();
Generate QR Code to Send SMS Text Message
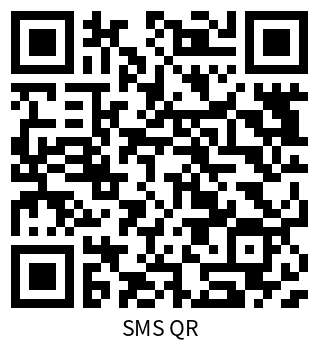
Now we will generate a QR code that will open up a Text Message and Pre-fill some information.
$qr_string = 'smsto:18888888888:Text Content Will Go Here;
$qrCode = QrCode::create($qr_string)
->setEncoding(new Encoding('UTF-8'))
->setErrorCorrectionLevel(new ErrorCorrectionLevelLow())
->setSize(300)
->setMargin(10)
->setRoundBlockSizeMode(new RoundBlockSizeModeMargin())
->setForegroundColor(new Color(0, 0, 0))
->setBackgroundColor(new Color(255, 255, 255));
$label = Label::create('Text Me')
->setTextColor(new Color(0, 0, 0));
$logo = null;
$result = $writer->write($qrCode, $logo, $label);
$dataUri = $result->getDataUri();
Generate Contact QR Code
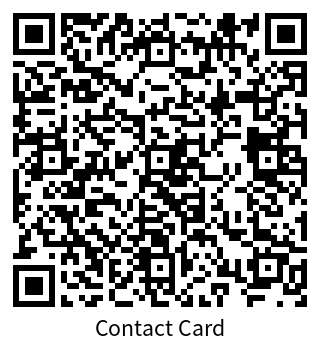
Now we will generate a QR code that will automatically add a Contact into a mobile device once scanned.
$name = 'My Name';
$mobile_num = '8888888888';
$phone_num = '8888888888';
$email = '[email protected]';
$company = 'CodeCr8 LLC';
$address = '123 Highway Rd';
$city = 'Some City';
$state = 'CA';
$zipcode = '99999';
$website = 'https://codecr8.com';
$qrContent = 'BEGIN:VCARD'."\n";
$qrContent .= 'VERSION:2.1'."\n";
$qrContent .= 'N:'.$name."\n";
$qrContent .= 'ORG:'.$company."\n";
$qrContent .= 'TEL;TYPE=cell:'.$mobile_num."\n";
$qrContent .= 'TEL;HOME;VOICE:'.$phone_num."\n";
$qrContent .= 'ADR;TYPE=work;'.
'Address:'
.$address.';'
.$city.';'
.$state.';'
.$zipcode
."\n";
$qrContent .= 'EMAIL:'.$email."\n";
$qrContent .= 'END:VCARD';
$qrCode = QrCode::create($qrContent)
->setEncoding(new Encoding('UTF-8'))
->setErrorCorrectionLevel(new ErrorCorrectionLevelLow())
->setSize(300)
->setMargin(10)
->setRoundBlockSizeMode(new RoundBlockSizeModeMargin())
->setForegroundColor(new Color(0, 0, 0))
->setBackgroundColor(new Color(255, 255, 255));
$label = Label::create('Add My Contact')
->setTextColor(new Color(0, 0, 0));
$logo = null;
$result = $writer->write($qrCode, $logo, $label);
$dataUri = $result->getDataUri();
Hope this was helpful in viewing the various ways to generate QR codes using Endroid QR Code library.
Take a look at our free qr code generator which you can use to generate all types of qr codes, download and use without any restrictions.